Origin of REST APIs
The Term "REST" (Representational State Transfer)
The term "REST" was coined by Roy Fielding in his doctoral thesis in 2000, titled "Architectural Styles and the Design of Network-based Software Architectures." Fielding, a prominent computer scientist who worked on the development of the HTTP/1.1 protocol and the creation of the Uniform Resource Identifier (URI) specification, introduced REST as an architectural style for designing distributed information systems on the World Wide Web.
REST is based on several key principles
- Resources: In REST, everything is a resource (objects or data), and each resource is uniquely identified by a Uniform Resource Locator (URL).
- CRUD Operations: CRUD operations (Create, Read, Update, Delete) are mapped to standard HTTP methods: POST, GET, PUT, and DELETE, respectively.
- Representational State: Resources are presented in machine-readable format, such as JSON or XML, and the application's state is transferred between the client and server.
- Statelessness: Each client request to the server must contain all the necessary information to understand and process the request, without depending on server-stored state.
- Stateless Interaction: Each client request to the server should be independent and not maintain client state on the server between requests.
- Resource Hierarchy: Resources can be hierarchically related, allowing for easier navigation through the API.
Differences Between REST and SOAP
SOAP (Simple Object Access Protocol)
SOAP is an XML-based communication protocol used to invoke methods and services over the network. Some key differences between REST and SOAP include:
- Protocol vs. Architectural Style: SOAP is a protocol, which means it defines specific rules and structures for requests and responses. REST is an architectural style that offers general guidelines for system design.
- Message Format: SOAP uses XML to format messages, which can be heavier and less human-readable. REST typically uses lighter, human-readable formats like JSON or XML.
- State Transfer: REST follows the "representational state" principle, where each request contains all necessary information. SOAP can maintain state on the server between requests.
- Transport: SOAP can use various transport protocols such as HTTP, SMTP, and more. REST commonly uses HTTP, making it simpler to implement and compatible with existing web infrastructure.
- Usage: SOAP has traditionally been used in enterprise environments and heavier web services, such as banking applications and enterprise resource planning systems. REST has become popular on the web and is widely used in web and mobile applications.
Advantages and Disadvantages of REST
Advantages of REST
- Simplicity: REST is easy to understand and use due to its web-based architectural style.
- Lightweight: Data formats like JSON are lighter and easier to process compared to XML (commonly used in SOAP).
- Scalability: REST is highly scalable and can handle growing traffic loads.
- Compatibility: Since it uses HTTP, REST is compatible with existing web infrastructure and can leverage its features.
Disadvantages of REST
- Lack of Standards: REST does not specify a strict set of standards, which can lead to a lack of consistency in implementation.
- Security: REST does not provide integrated security mechanisms, requiring additional measures like OAuth for authentication and authorization.
- Less Functionality: Compared to SOAP, REST may lack some advanced features such as ACID transactions and advanced error handling.
Good practices
1- Use of Meaningful Names
- Clear Resource Names: Resource names (URLs) should be descriptive and meaningfully represent the entity or resource being manipulated. For example, "/users" instead of "/data."
- Plural Route Names: Use plural route names to represent collections, for example, "/users" instead of "/user."
2- Versioning
- Explicit Versioning: Include the API version in the URL to ensure future compatibility. For example, "/v1/users."
- Gradual Migration: If a significant change is made to the API, provide a transition period during which both the old and new versions are supported.
3- HTTP Methods
- Proper Use of HTTP Methods: Use standard HTTP methods appropriately, such as GET (for retrieving resources), POST (for creating resources), PUT (for updating resources), and DELETE (for deleting resources).
- Idempotent Methods: Ensure that PUT and DELETE methods are idempotent, meaning that multiple calls have the same result as a single call.
4- Responses and Status Codes
- Appropriate Status Codes: Use appropriate HTTP status codes to indicate the outcome of an operation (e.g., 200 OK, 201 Created, 204 No Content, 400 Bad Request, 404 Not Found, etc.).
- Informative Responses: Provide informative JSON responses with details about the request's result, especially in case of errors.
5- Data Structure
- JSON as Default Format: Use JSON as the default data exchange format due to its readability and compatibility.
- Consistent Data Structure: Maintain a consistent data structure in all responses and requests to allow clients to predict the data structure.
6- Use of HTTP Headers
- Proper Headers: Use HTTP headers to provide additional information, such as authentication (using the Authorization header), pagination (using Link or X-Pagination), and content (using Content-Type and Accept).
7- Authentication and Authorization
- Secure Authentication: Use secure authentication methods such as OAuth 2.0 or JSON Web Tokens (JWT) to protect your endpoints.
- Role-Based Authorization: Implement a role-based authorization system to limit access to sensitive resources.
8- Pagination and Sorting
- Pagination: Implement pagination when responses contain large datasets to allow clients to retrieve data efficiently.
- Sorting: Provide sorting options in requests to allow clients to order results as needed.
9- Error Handling
- Detailed Error Messages: Provide clear and detailed error messages in response bodies to help developers troubleshoot issues.
- Standard Error Codes: Define a set of standard error codes so that clients can interpret and handle errors consistently.
10- Documentation
- Comprehensive Documentation: Provide comprehensive API documentation that includes usage examples, resource descriptions, request and response examples, and authentication examples.
- Documentation Tools: Use tools like Swagger or Postman to generate interactive API documentation.
11- Testing and Monitoring
- Thorough Testing: Conduct comprehensive API testing, including unit tests, integration tests, and performance tests.
- Continuous Monitoring: Implement a monitoring solution to monitor API performance and availability in real-time.
12- Security
- Protection Against Attacks: Implement security measures, such as preventing SQL injection, Cross-Site Request Forgery (CSRF), and Cross-Site Scripting (XSS) attacks.
- CORS (Cross-Origin Resource Sharing): Configure CORS properly to control access to the API from different domains.
13- Version Control and Lifecycle
- Version Control: Use version control systems to manage the API's source code and ensure updates are controlled.
- API Lifecycle: Establish a clear API lifecycle that includes development, testing, deployment, and retirement phases.
14- Scalability
- Scalable Design: Design the API to be horizontally scalable to handle increasing traffic loads.
- Caching: Use caching strategies to reduce server load and improve performance.
15- Logging and Auditing
- Detailed Logs: Keep detailed logs of all activities in the API for auditing and troubleshooting purposes.
- Access Auditing: Implement access auditing to track who accesses the API and what actions they perform.
16- Security Testing
- Regular Security Testing: Conduct regular security testing, such as penetration testing, to identify potential vulnerabilities and security risks.
- Security Updates: Keep all API components up to date and apply security patches as needed.
17- Performance Monitoring
- Performance Monitoring Tools: Use performance monitoring tools to identify bottlenecks and optimize the API accordingly.
- Resource Tuning: Adjust underlying resources (databases, servers, caching) for optimal performance.
18- Backward Compatibility
- Backward Compatibility: If possible, maintain backward compatibility with older API versions to minimize disruption to existing clients.
- Planned Deprecation: Announce planned deprecation of older versions in advance and provide a transition period.
19- Developer Communication
- Clear Communication: Establish clear communication channels with client developers, such as forums, emails, or chat, to address questions and provide support.
- Change Notifications: Inform developers about significant API changes and planned updates.
20- Network Optimization
- Data Compression: Use data compression (e.g., GZIP) to reduce response size and improve data transfer efficiency.
- Content Delivery Network (CDN): Consider using a CDN to distribute static content and reduce latency.
Structure basic for Express.JS
src/
The "src" (source) folder contains all the source code of the application. This is where the main logic of your API is located.
controllers/
This folder stores controllers that handle incoming HTTP requests and process the associated business logic for those requests. For example, the "userController.js" could handle requests related to users.
models/
Here, data models are defined to represent the entities or resources of your application. For instance, if your API is about users, you could have a file like "User.js" that defines the user model.
routes/
This folder is used to define the API routes and configure middleware. The "api.js" file typically contains the main routes of the API and how requests should be handled.
services/
This folder is optional and can be used to organize services related to the business logic of your application. Services can contain functions and operations that are used in multiple controllers or parts of the application.
app.js
This is the main application file. It's where routes are configured, the web server is started, and the primary application configuration takes place.
config.js
This file stores the application's configuration, such as database configuration, authentication settings, and other configuration variables.
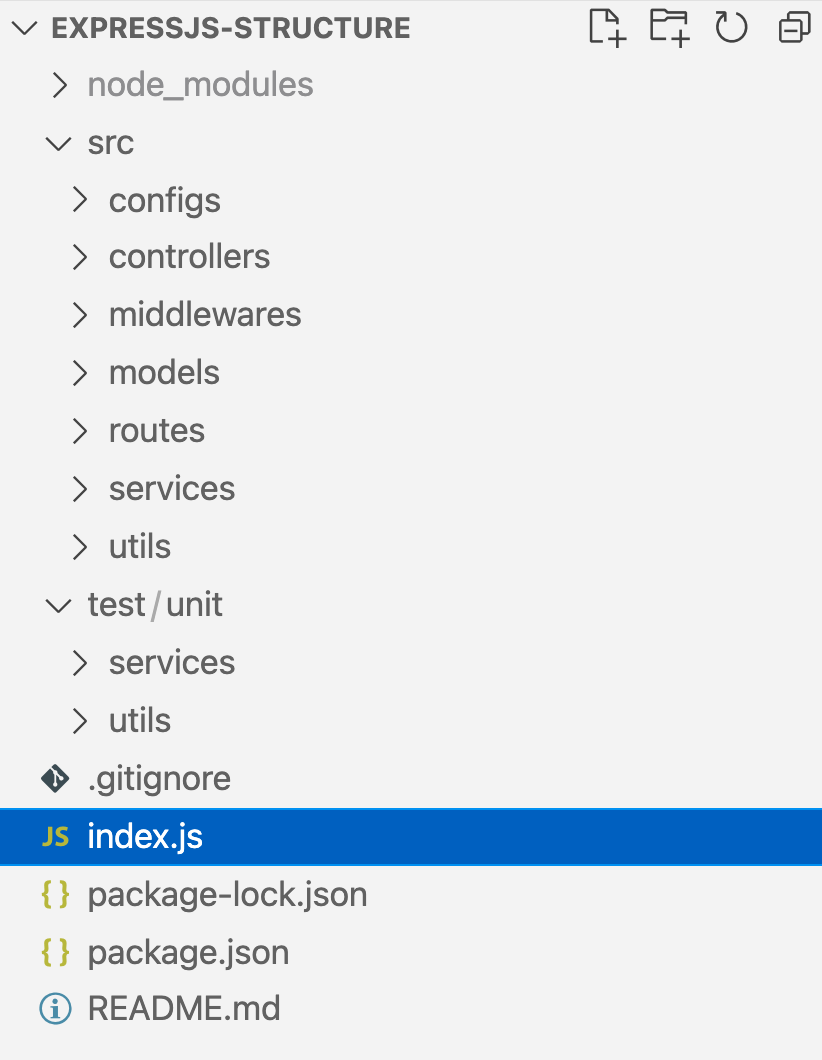
